더보기
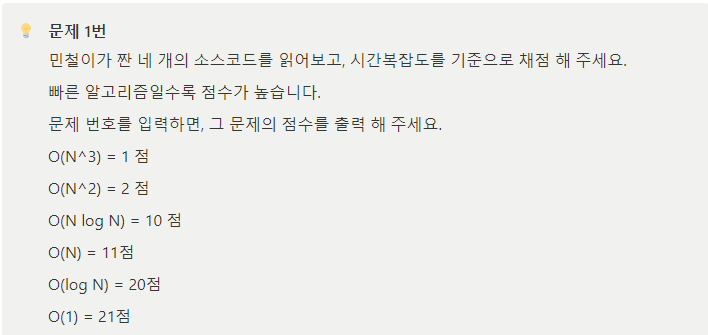
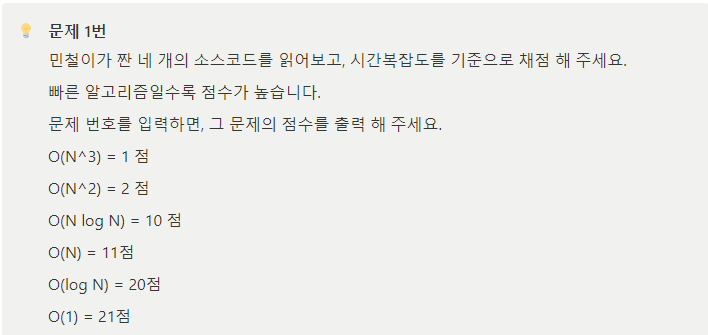
#include <iostream>
using namespace std;
int main()
{
// 알고리즘 1. O(1)
{
/*#include <iostream>
using namespace std;
int main()
{
for (int i = 0; i < 10000; i++) {
cout << "#";
}
return 0;
}*/
}
// 알고리즘 2. O(n)
{
/*#include <iostream>
using namespace std;
int main()
{
int n;
cin >> n;
for (int y = 0; y < n; y++) {
for (int x = 0; x <= y; x++) {
cout << "#";
}
}
return 0;
}*/
}
// 알고리즘 3. O(n^2)
{
/*#include <iostream>
using namespace std;
int n;
void abc()
{
for (int i = 0; i < n; i++) {
cout << "#";
}
}
int main()
{
cin >> n;
for (int y = 0; y < n; y++) {
abc();
abc();
abc();
}
return 0;
}*/
}
//알고리즘 4. O(n^2)
{
/*#include <iostream>
using namespace std;
int main()
{
cin >> n;
for (int y = 0; y < n; y++) {
for (int x = 0; x < 5; x++) {
for (int z = 0; z < n; z++) {
cout << "#";
}
}
}
return 0;
}*/
}
int arr[5] = { -1, 21, 11, 2, 2 };
int algo;
cin >> algo;
int score = arr[algo];
return 0;
}
더보기
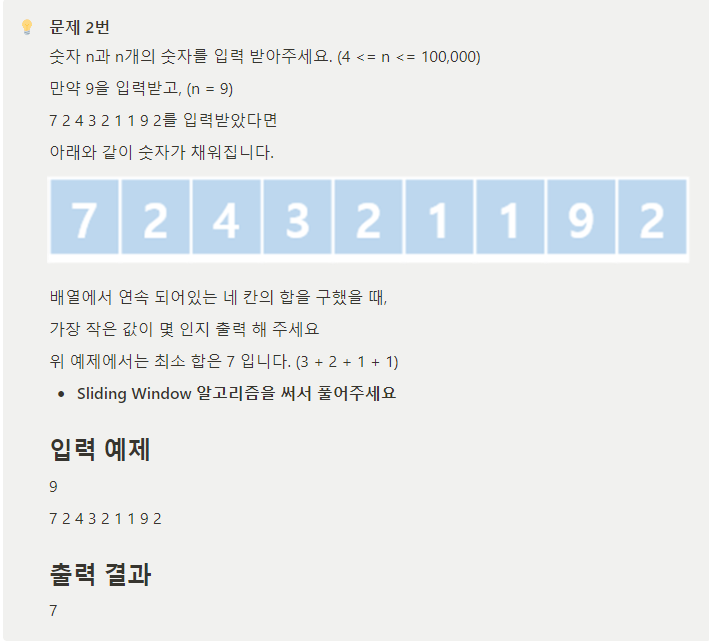
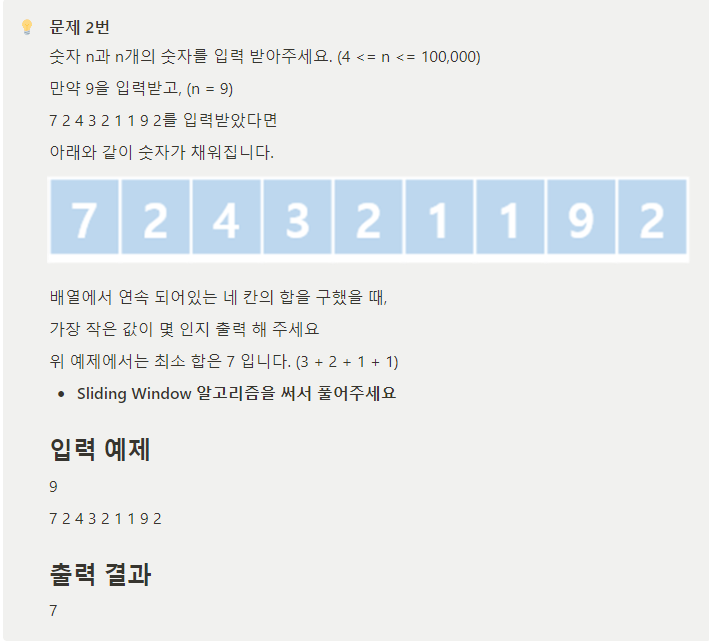
#include <iostream>
using namespace std;
int main()
{
const int MAX = 100000;
int arr[MAX] = { 7, 2, 4, 3, 2, 1, 1, 9, 2 };
int n = 9;
while (true)
{
cin >> n;
if (n >= 4 && n <= MAX)
{
for (int i = 0; i < n; i++)
{
cin >> arr[i];
}
break;
}
}
int min = INT_MAX;
int length = (n - 4) + 1;
int sum = 0;
for (int i = 0; i < 4; i++)
{
sum += arr[i];
}
for (int i = 1; i < length; i++)
{
sum = sum - arr[i - 1] + arr[i + 3];
if (min > sum)
min = sum;
}
return 0;
}
더보기
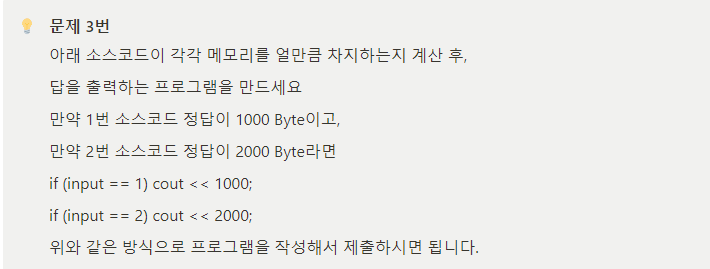
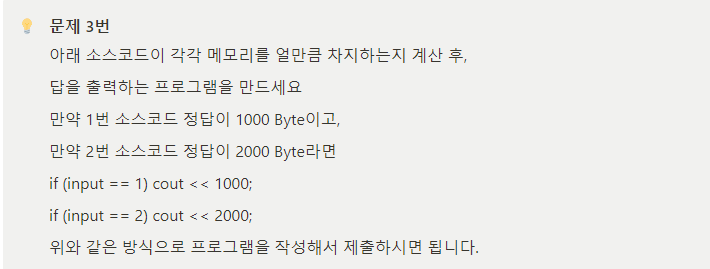
#include <iostream>
using namespace std;
int main()
{
// 1번 40 byte
{
/*#include <iostream>
using namespace std;
int data[10];
int main()
{
return 0;
}*/
}
// 2번 24 + 10 + 40
{
/*#include <iostream>
using namespace std;
double data[3]; 8 24
char vect[10]; 1 10
int dt[10]; 4 40
int main()
{
return 0;
}*/
}
// 3번 800
{
/*#include <iostream>
using namespace std;
struct Node
{
int x; 4
char t; 1 + 3;
};
Node vect[100]; 800
int main()
{
return 0;
}*/
}
// 4번 16
{
/*#include <iostream>
using namespace std;
struct Node { int x; char* next; };
Node vect;
int main() { return 0; }*/
}
int input = 0;
cin >> input;
if (input == 1)
cout << 40;
if (input == 2)
cout << 74;
if (input == 3)
cout << 800;
if (input == 4)
cout << 16;
return 0;
}
더보기
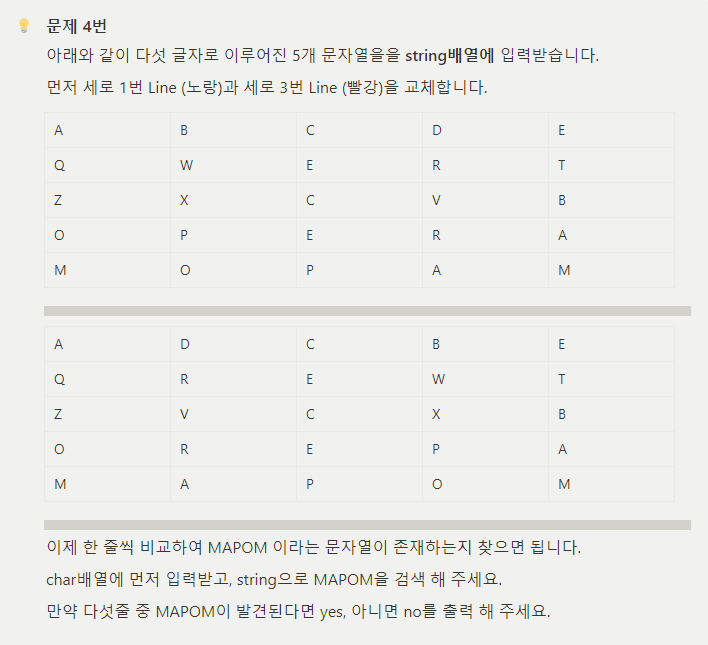
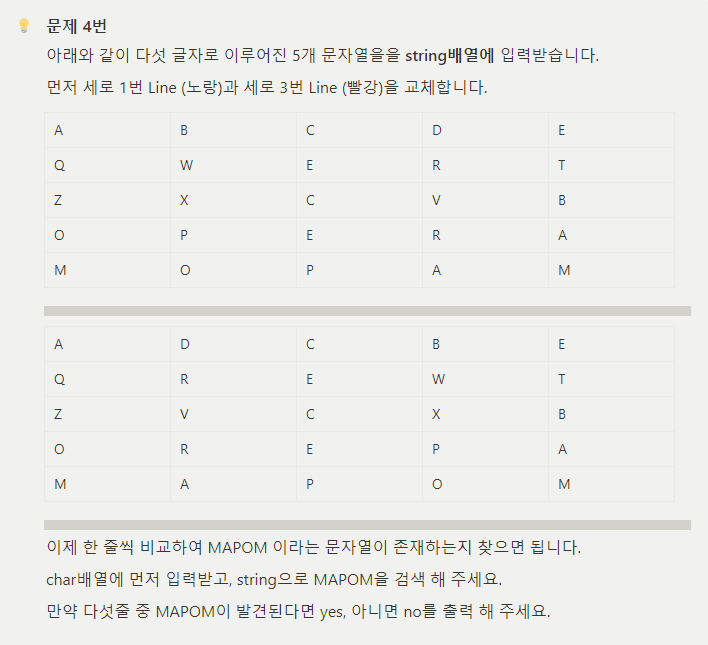
#include <iostream>
using namespace std;
const int num = 5;
string str[5];
string MAPOM = "MAPOM";
void SwapChar()
{
const int line1 = 1;
const int line3 = 3;
for (int i = 0; i < num; i++)
{
char temp = str[i][line1];
str[i][line1] = str[i][line3];
str[i][line3] = temp;
}
}
void SearchSRT()
{
for (int i = 0; i < num; i++)
{
if (MAPOM == str[i])
{
cout << "yes";
return;
}
}
cout << "no";
}
int main()
{
for (int i = 0; i < num; i++)
{
cin >> str[i];
}
SwapChar();
SearchSRT();
return 0;
}
더보기
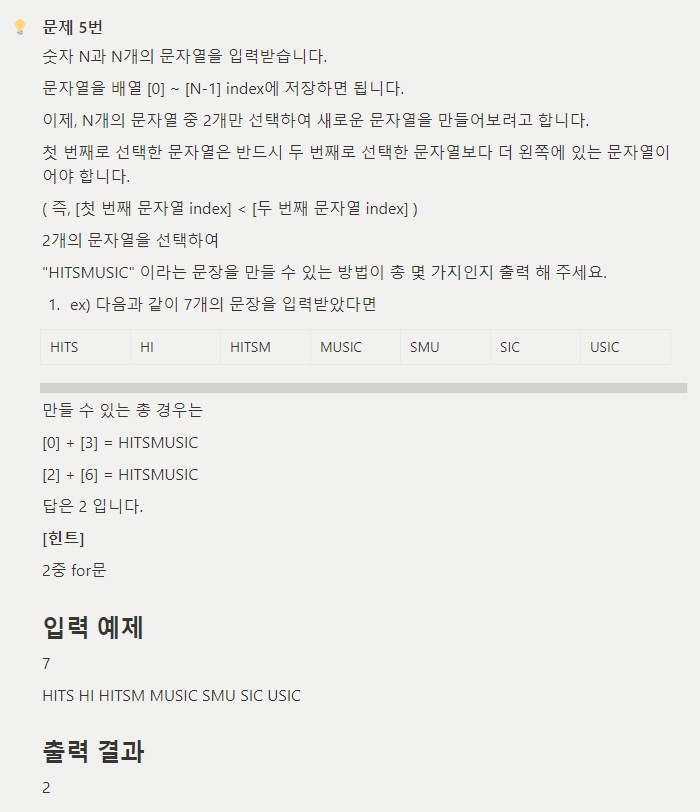
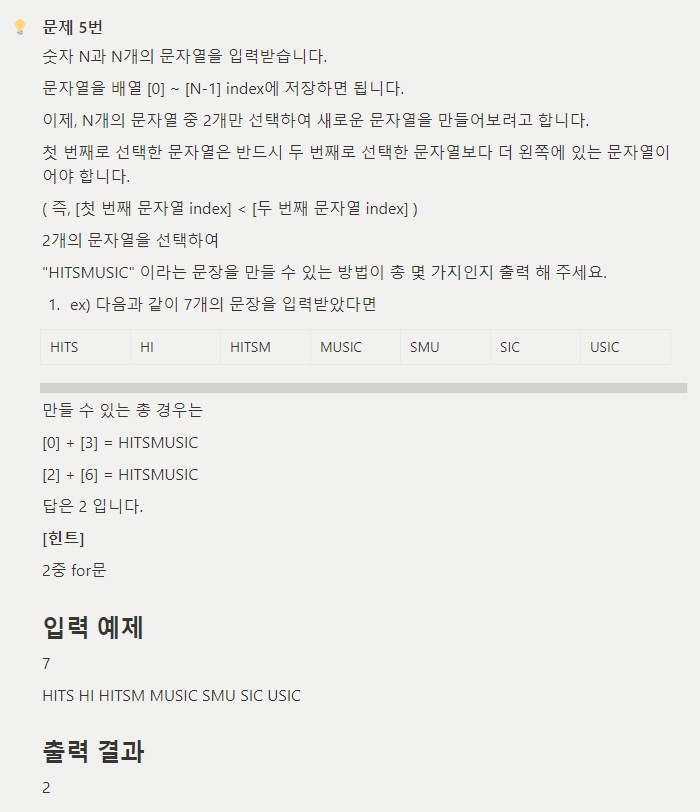
#include <iostream>
using namespace std;
const string HITSMUSIC = "HITSMUSIC";
string arr[100];
int main()
{
int N;
cin >> N;
for (int i = 0; i < N; i++)
{
cin >> arr[i];
}
int count = 0;
for (int i = 0; i < N; i++)
{
for (int j = i + 1; j < N; j++)
{
string add = arr[i] + arr[j];
if (HITSMUSIC == add)
count++;
}
}
return 0;
}
더보기
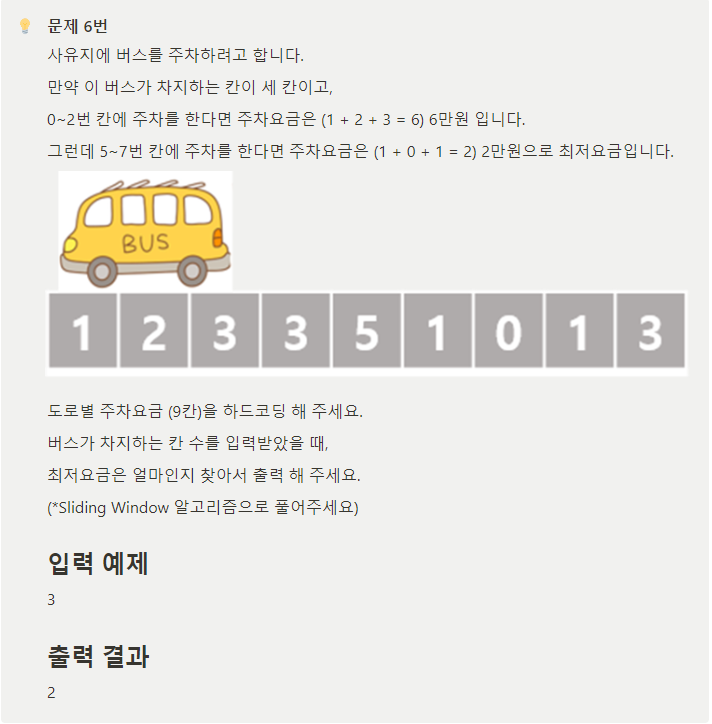
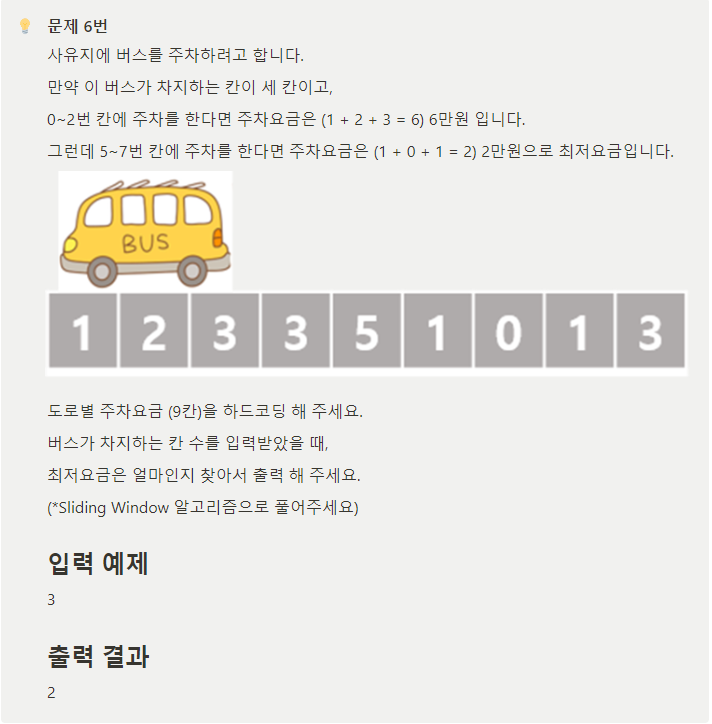
#include <iostream>
using namespace std;
int main()
{
int cost[9] = { 1,2,3,3,5,1,0,1,3 };
int busSize;
int length;
int sum = 0;
cin >> busSize;
length = 9 - busSize + 1;
for (int i = 0; i < busSize; i++)
{
sum += cost[i];
}
int min = INT_MAX;
for (int i = 1; i < length; i++)
{
sum = sum - cost[i - 1] + cost[i + busSize - 1];
if (min > sum)
min = sum;
}
return 0;
}
더보기
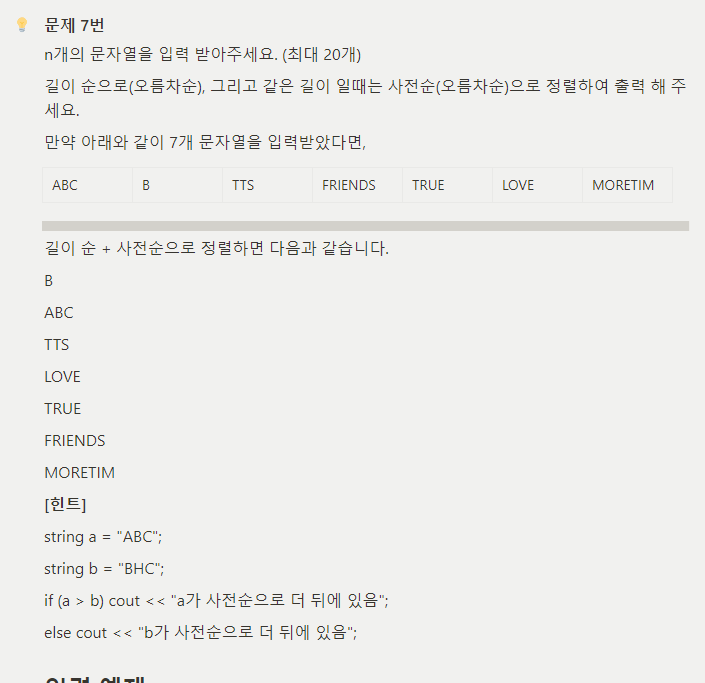
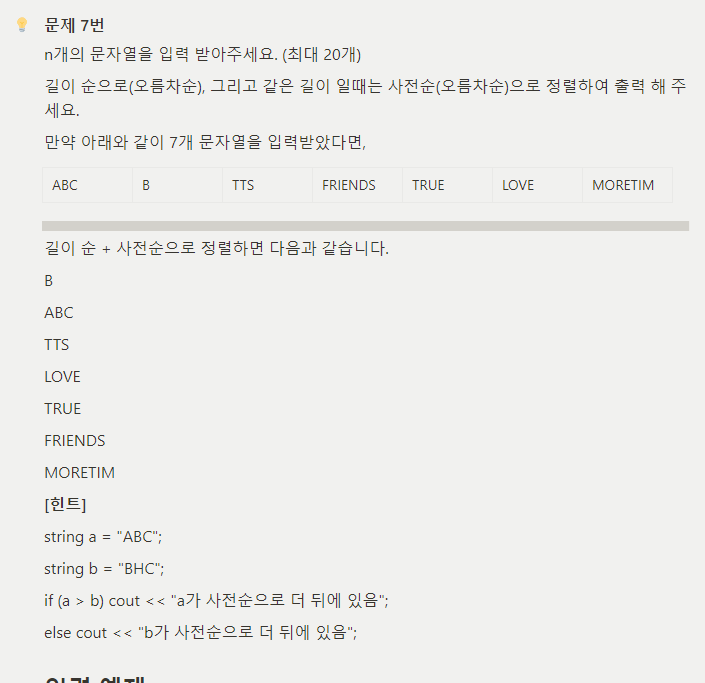
#include <iostream>
using namespace std;
int main()
{
int n;
string str[20];
cin >> n;
for (int i = 0; i < n; i++)
{
cin >> str[i];
}
for (int i = 0; i < n; i++)
{
for (int j = i + 1; j < n; j++)
{
if (str[i].length() > str[j].length())
{
string temp = str[i];
str[i] = str[j];
str[j] = temp;
}
else if (str[i].length() == str[j].length())
{
if (str[i] > str[j])
{
string temp = str[i];
str[i] = str[j];
str[j] = temp;
}
}
}
}
return 0;
}
더보기
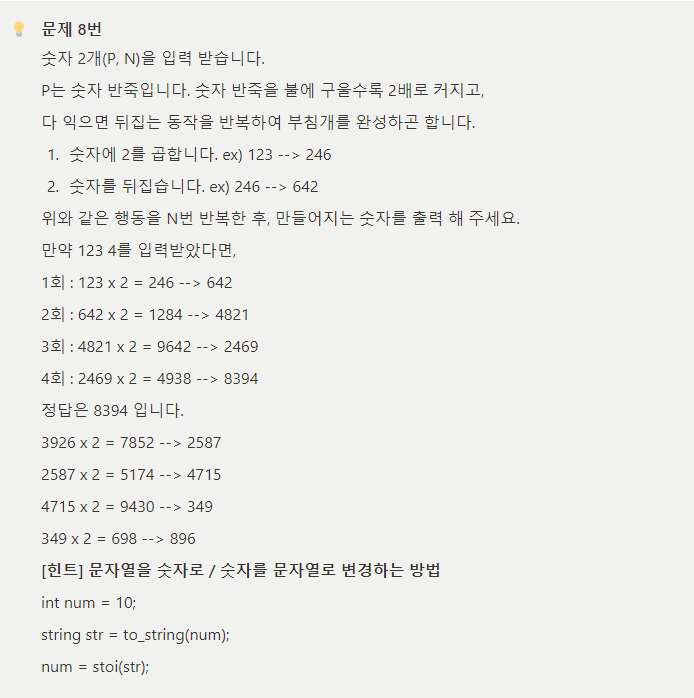
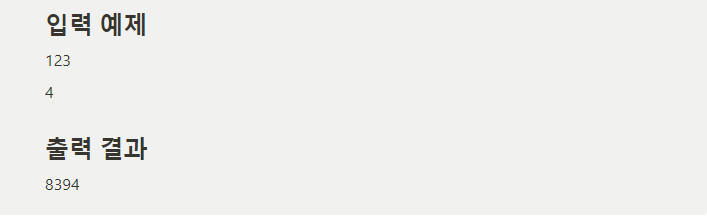
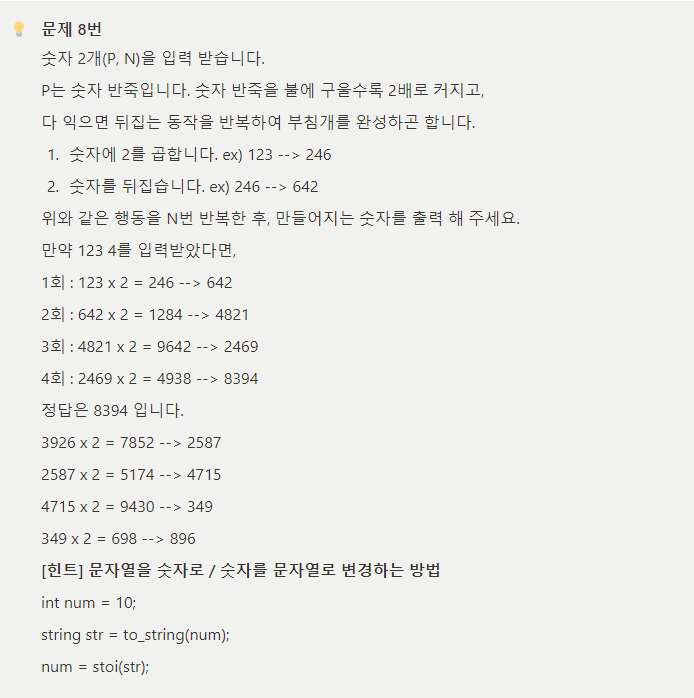
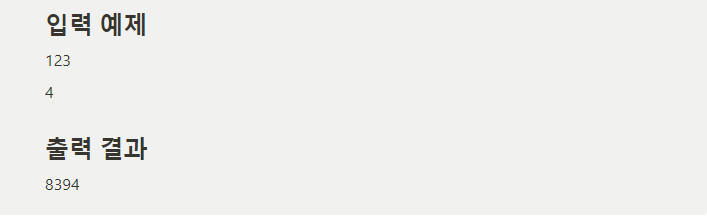
#include <iostream>
#include <string>
using namespace std;
int main()
{
int P;
int N;
cin >> P >> N;
for (int i = 0; i < N; i++)
{
int mult = P * 2;
string str = to_string(mult);
int front = 0;
int tail = static_cast<int>(str.length() - 1);
for (int j = 0; j < str.length(); j++)
{
if ((front + j) >= (tail - j))
{
P = stoi(str);
break;
}
char temp = str[front + j];
str[front + j] = str[tail - j];
str[tail - j] = temp;
}
}
return 0;
}
더보기
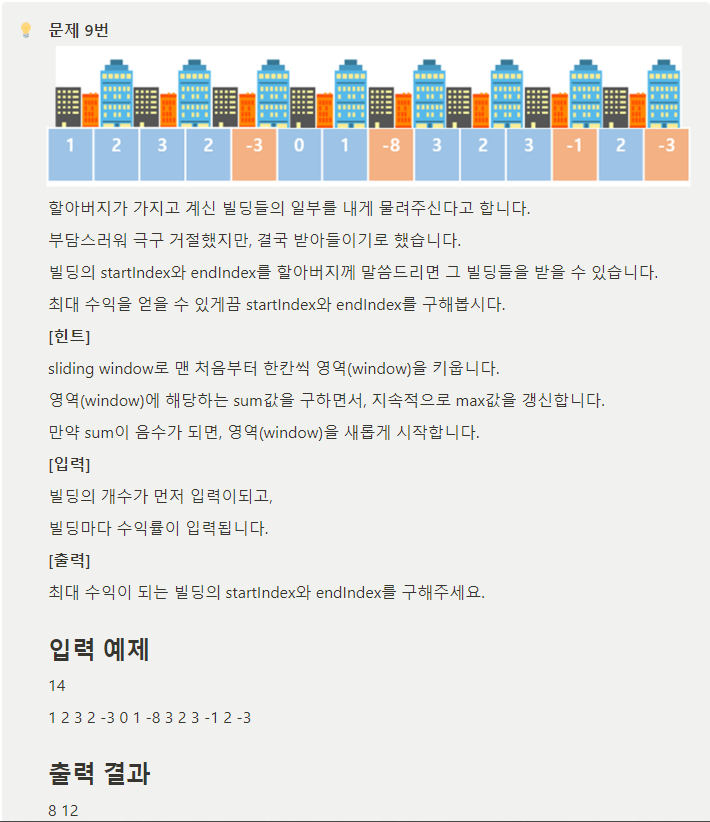
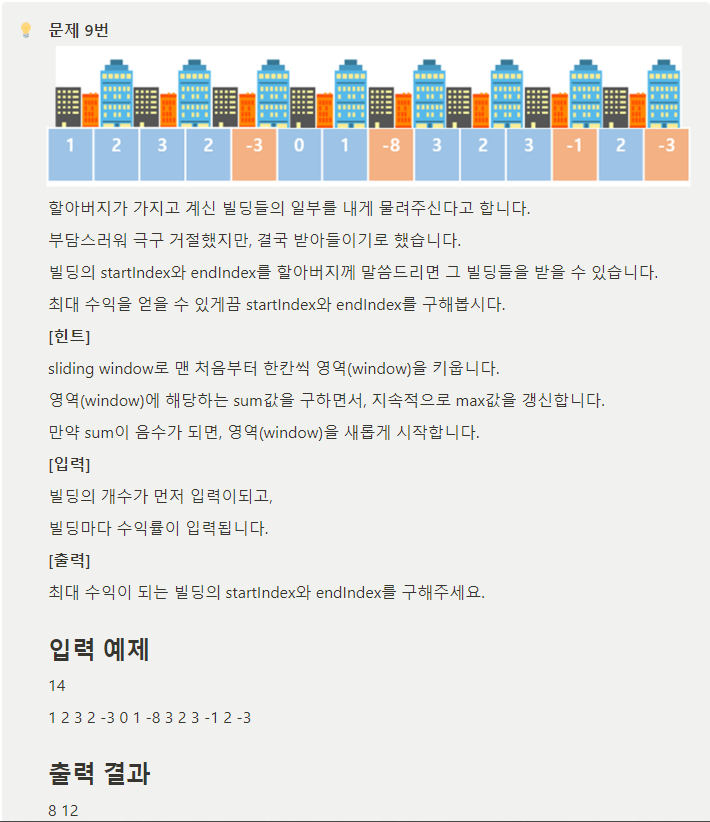
#include <iostream>
#include <vector>
using namespace std;
int main()
{
int amount;
vector<int> cost;
cin >> amount;
for (int i = 0; i < amount; i++)
{
int a;
cin >> a;
cost.push_back(a);
}
int startIndex = 0;
int endIndex = 0;
int max = -1;
for (int i = 0; i < amount; i++)
{
int sum = 0;
sum += cost[i];
for (int j = i + 1; j < amount; j++)
{
sum += cost[j];
if (sum < 0)
break;
if (sum > max)
{
max = sum;
startIndex = i;
endIndex = j;
}
}
}
return 0;
}